Ensure data consistency
Benefit from the reusability and modularity of TypeSpec types to ensure data consistency across your APIs.
Get started import "./common.tsp";
namespace MyOrg.Accounts;
using MyOrg.Types;
model Account { id: id; firstName: string; lastName: string; createdAt: utcDateTime;
// Use imported type by name only when using `using` ssn: ssn;
// Or use the fully qualified name email: MyOrg.Types.email;
balance: Amount;}
model Amount { value: decimal128; currency: Currency;}
// Create your own error types by extending the Error typemodel AccountError is Error<"duplicate-account" | "invalid-account">;
op createAccount(account: Account): Account;
op charge(accountId: id, amount: Amount): void | AccountError;
namespace MyOrg.Types;
@format("uuid")scalar uuid extends string;
// Defined a standard id type that all models can usescalar id extends uuid;
@pattern(".+\\@.+\\..+")scalar email extends string;
@pattern("^\\d{3}-\\d{2}-\\d{4}$")scalar ssn extends string;
/** * Standard error response */model Error<T extends string> { code: T; message: string; details?: Record<string>; timestamp: utcDateTime;}
enum Currency { USD, EUR, GBP, CAD, JPY,}
Standard library
Use built-in decorators
TypeSpec standard library provides decorators for common validation patterns.
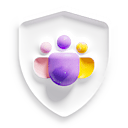
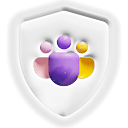
Standard library reference
Browse the standard library reference documentation for details.
Learn more →
model User { @minLength(3) @maxLength(50) username: string;
@secret password: string;
@minValue(0) @maxValue(200) age: uint32;
@minItems(1) @maxItems(10) emails: string[];}
@pattern(".+\\@.+\\..+")scalar email extends string;
Output
Produce JSON Schema
Benefit from the JSON Schema ecosystem to validate your data while writing more concise and readable code.
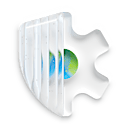

Configure the JSON schema emitter
Change how the JSON schema is emitted: specify a bundleId to combine all schemas into a single file or use JSON instead of yaml.
Learn more →
import "@typespec/json-schema";
using TypeSpec.JsonSchema;
@jsonSchemanamespace Schemas;
model Person { /** The person's full name. */ name: string;
/** Person address */ address: Address;
/** List of nick names */ @uniqueItems nickNames?: string[];
/** List of cars person owns */ cars?: Car[];}
/** Respresent an address */model Address { street: string; city: string; country: string;}
model Car { /** Kind of car */ kind: "ev" | "ice";
/** Brand of the car */ brand: string;
/** Year the car was manufactured. */ @minValue(1900) year: int32;}
$schema: https://json-schema.org/draft/2020-12/schema$id: Address.yamltype: objectproperties: street: type: string city: type: string country: type: stringrequired: - street - city - countrydescription: Respresent an address
$schema: https://json-schema.org/draft/2020-12/schema$id: Car.yamltype: objectproperties: kind: anyOf: - type: string const: ev - type: string const: ice description: Kind of car brand: type: string description: Brand of the car year: type: integer minimum: 1900 maximum: 2147483647 description: Year the car was manufactured.required: - kind - brand - year
$schema: https://json-schema.org/draft/2020-12/schema$id: Person.yamltype: objectproperties: name: type: string description: The person's full name. address: $ref: Address.yaml description: Person address nickNames: type: array items: type: string uniqueItems: true description: List of nick names cars: type: array items: $ref: Car.yaml description: List of cars person ownsrequired: - name - address
Customize
JSON Schema Decorators
The JSON schema library provides decorators to customize the output with JSON schema specific concepts.
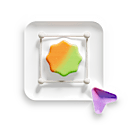
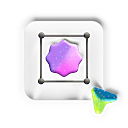
JSON Schema Decorators Reference
Read the reference documentation for available options.
Learn more →
import "@typespec/json-schema";
using TypeSpec.JsonSchema;
@jsonSchema@extension( "x-tag", Json<{ validate: true, category: "users", }>)model Server { @format("hostname") hostname: string;}
$schema: https://json-schema.org/draft/2020-12/schema$id: output.yaml$defs: Server: $schema: https://json-schema.org/draft/2020-12/schema $id: Server type: object properties: hostname: type: string format: hostname required: - hostname x-tag: validate: true category: users