Write TypeSpec, emit OpenAPI
Emitting OpenAPI from TypeSpec enables seamless cross-language interaction, automates API-related tasks, and simplifies API management and evolution.
Get started import "@typespec/http";
using TypeSpec.Http;
model Pet { name: string; age: int32;}
model Store { name: string; address: Address;}
model Address { street: string; city: string;}
@route("/pets")interface Pets { list(@query filter: string): Pet[]; create(@body pet: Pet): Pet; read(@path id: string): Pet;}
@route("/stores")interface Stores { list(@query filter: string): Store[]; read(@path id: string): Store;}
openapi: 3.0.0info: title: (title) version: 0.0.0tags: []paths: /pets: get: operationId: Pets_list parameters: - name: filter in: query required: true schema: type: string responses: '200': description: The request has succeeded. content: application/json: schema: type: array items: $ref: '#/components/schemas/Pet' post: operationId: Pets_create parameters: [] responses: '200': description: The request has succeeded. content: application/json: schema: $ref: '#/components/schemas/Pet' requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/Pet' /pets/{id}: get: operationId: Pets_read parameters: - name: id in: path required: true schema: type: string responses: '200': description: The request has succeeded. content: application/json: schema: $ref: '#/components/schemas/Pet' /stores: get: operationId: Stores_list parameters: - name: filter in: query required: true schema: type: string responses: '200': description: The request has succeeded. content: application/json: schema: type: array items: $ref: '#/components/schemas/Store' /stores/{id}: get: operationId: Stores_read parameters: - name: id in: path required: true schema: type: string responses: '200': description: The request has succeeded. content: application/json: schema: $ref: '#/components/schemas/Store'components: schemas: Address: type: object required: - street - city properties: street: type: string city: type: string Pet: type: object required: - name - age properties: name: type: string age: type: integer format: int32 Store: type: object required: - name - address properties: name: type: string address: $ref: '#/components/schemas/Address'
Ecosystem
Interoperate with the OpenAPI ecosystem
Benefit from a huge ecosystem of OpenAPI tools for configuring API gateways, generating code, and validating your data.

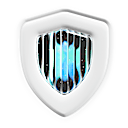
Linters
Integrate with spectral to lint your OpenAPI.
Learn more →

@service({ title: "Pet Store Service",})namespace PetStore;
op ping(): void;
openapi: 3.0.0info: title: Pet Store Service version: 2021-03-25tags: []paths: /: get: operationId: ping parameters: [] responses: "204": description: "There is no content to send for this request, but the headers may be useful. "components: {}
data:text/plain;base64,W3NlY29uZGFyeV1+ICQgWy9zZWNvbmRhcnldIHNwZWN0cmFsIGxpbnQgb3BlbmFwaS55YW1sCgpbYnJhbmQudW5kZXJsaW5lXS9vcGVuYXBpLnlhbWxbL2JyYW5kLnVuZGVybGluZV0KIDE6MSAgW3dhcm5pbmddd2FybmluZ1svd2FybmluZ10gIG9hczMtYXBpLXNlcnZlcnMgICAgICAgT3BlbkFQSSAic2VydmVycyIgbXVzdCBiZSBwcmVzZW50IGFuZCBub24tZW1wdHkgYXJyYXkuCiAyOjYgIFt3YXJuaW5nXXdhcm5pbmdbL3dhcm5pbmddICBpbmZvLWNvbnRhY3QgICAgICAgICAgIEluZm8gb2JqZWN0IG11c3QgaGF2ZSAiY29udGFjdCIgb2JqZWN0LiAgICAgICAgICAgICAgICAgICAgICAgIGluZm8KIDI6NiAgW3dhcm5pbmddd2FybmluZ1svd2FybmluZ10gIGluZm8tZGVzY3JpcHRpb24gICAgICAgSW5mbyAiZGVzY3JpcHRpb24iIG11c3QgYmUgcHJlc2VudCBhbmQgbm9uLWVtcHR5IHN0cmluZy4gICAgICAgaW5mbwogODo5ICBbd2FybmluZ113YXJuaW5nWy93YXJuaW5nXSAgb3BlcmF0aW9uLWRlc2NyaXB0aW9uICBPcGVyYXRpb24gImRlc2NyaXB0aW9uIiBtdXN0IGJlIHByZXNlbnQgYW5kIG5vbi1lbXB0eSBzdHJpbmcuICBwYXRocy4vLmdldAogODo5ICBbd2FybmluZ113YXJuaW5nWy93YXJuaW5nXSAgb3BlcmF0aW9uLXRhZ3MgICAgICAgICBPcGVyYXRpb24gbXVzdCBoYXZlIG5vbi1lbXB0eSAidGFncyIgYXJyYXkuICAgICAgICAgICAgICAgICAgICBwYXRocy4vLmdldAo=
Ecosystem
Abstract recurring patterns
Transform API patterns into reusable elements to enhance both the quality and uniformity of your API interface.
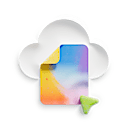
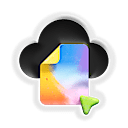
Example: TypeSpec Azure Library
The TypeSpec library for Azure allows multiple teams to reuse pre-approved patterns.
Learn more →
import "@typespec/http";
using TypeSpec.Http;
// Define abstraction for resource lifecyleop ResourceList<T>(@query filter: string): T[];op ResourceRead<T>(@path id: string): T;@post op ResourceCreate<T>(...T): T;
model Pet { name: string; age: int32;}
@route("/pets")interface Pets { list is ResourceList<Pet>; create is ResourceCreate<Pet>; read is ResourceRead<Pet>;}